Nuance Messaging UI Framework
Nuance Messaging UI framework exposes a fully functional Messaging UI viewController that can be launched from your application. Framework exposes custom UI properties which allows App developer to customize the look and feel of the Messaging UI.
- Launching ViewController
- Plain Bubble View
- Styling Send Button
- Styling Customer Input Field
- Styling Footer Separation Bar
- Styling Title Bar
- Styling Transcript View
- Styling Transcript bubbles using background Image
- Styling Speech bubbles view
- Styling Email Dialog
- Styling Loading View
- Messaging App String Properties
- Displaying customer/Agent name in bubbles
- Displaying and styling timestamp
- Styling File Upload screen
- Customizing VA resposne
- Displaying disclaimer header
- Display Survey Confirmation Dialog
- Additional Typing Customization
- Hidden messages
Nuance UI Framework exposes methods to initiate a new engagement session aswell as to restore an existing engagement session.
public func launchMessagingViewController(params: EngageParameters)
params: Engage Paramters needed to create an engagement.
siteID | SiteID parameter is mandatory to create an engagement. |
businessUnitID | Mandatory Business Unit ID to check agent availability and HOP availability. |
agentGroupID | Mandatory AgentGroupID which is required for a new engagement. |
businessRuleID | Mandatory Business rule ID is used to tie this engagement to a business rule for reporting purposes |
openerText | Opener message that will be added to transcript upon agent assign. |
opID | ID of the Opener message that is configure is portal. |
brName | BusinessRuleName parameter is optional. |
autoID | Associated automation ID with this engagement request. |
pageID | Page Marker ID where an engagement starts. |
prioity | Initial engagement priority of the engagement request. A higher number indicates a higher priority in the queue |
qmSpecID | The ID of the queue messaging spec as identified in TouchPortal. If the queue messaging spec is valid, it will pull the proper queue message and display it as message text when the API caller calls the Get message API call. |
qt | Queue threshold value for engagement. Used to calculate whether this engagement goes into the queue in cases where agent slots are not available. |
scriptID | ID of the agent’s scripts for this engagement. |
dataPass | Datapass information for this engagement in name value pair. |
agentAttributes | Agent attribute information used for this engagement. |
isAsyncEngagement | Set the value to true if this is an asyncronus engagement. |
customerName | Value of this parameter will be displayed in the customer bubble. Refer "Displaying Customer/Agent name in bubbles" section of this page |
autoDataPass | Use this field to pass automaton Datapass. |
agentAssistedCount | Use this field to set the minimum number of agent message to be qualified for assisted criteria. |
customerAssistedCount | Use this field to set the minimum number of customer message to be qualified for assisted criteria. |
pcs_agent_count | Use this field to set the minimum number of agent message to be qualified for post chat survey. |
pcs_customer_count | Use this field to set the minimum number of customer message to be qualified for post chat survey. |
emailSpecID | Use this parameter to configure the email spec settings. |
public func restoreMessagingViewController(webview: WKWebView?)
webView parameter is optional and only need to be passed if there is a webview involved in this engagement request.
public func launchMessagingViewControllerForHybrid(paramsDict: Dictionary
This method must be used when engagement is requested from the Nuance BR JS engine running within webView page
paramsDict: Pass the engageparameter dictionary provided by the handleWebviewRequest andNewEngagementHandler callback(refer hybrid section of this document)
Below Function lets you close the messaging window from outside the SDK. This method is accessible from the instance returned from launchMessagingViewController
closeAndDismiss()
Below Function lets you minimize the messaging window from outside the SDK. This method is accessible from the instance returned from launchMessagingViewController
minimizeScreen()
Below Function lets you change the title after an engagement is created.This method is accessible from the instance returned from launchMessagingViewController
updateMessagingTitle()
Below Function lets you change the logo after an engagement is created.This method is accessible from the instance returned from launchMessagingViewController
updateMessagingLogo()
Below Function lets application to display a typing mesage /animation programatically.This method is accessible from the instance returned from launchMessagingViewController
displayAgentTyping()
Below Function lets application to add a message on to the chat window programatically.This method is accessible from the instance returned from launchMessagingViewController
addMessageToChatWindow()
Below Function lets application to add a system message on to the chat window programatically.This method is accessible from the instance returned from launchMessagingViewController
addSystemToChatWindow()
Below Function lets application to clear a system message.This method is accessible from the instance returned from launchMessagingViewController
clearSystemFromChatWindow()
Below Function lets application to add a custom VA message on to the chat window programatically.This method is accessible from the instance returned from launchMessagingViewController
addCustomVAMsg()
Below Function lets you check the state of the chat.
NuanMessaging.getInstance().getChatProgress()
Below Function lets you check the SDK version.
NuanMessaging.getVersion()
Below Function to set the SDK timeout for an active engagement.Engagement can be timed due to application being in background or messaging window is minimized but application is not pooling for agent messages when minimized. This timeout should be less than or equal to chat router timeout set in portal
NuanMessaging.getInstnace().setEngagementTimeoutInSeconds()
Below Function lets you Override the nuance domain SDK is pointing to. This must be called prior to the call to initialize method.
NuanMessaging.getInstance()?.overrideAPIDomain()
NuanMessaging.getInstance()?.overrideAuthDomain()
By passing an instance of NuanceMessagingDelegate class to below method lets application to listen to various SDK events.
public func setMessagingDelegate(delegate: Any)
onMinimized(): SDK fires this method when Messaging Window is minimized.
onClosed(): SDK fires this method when Messaging Window is closed.
onAgentAssigned(): SDK fires this method when an agent is assigned.
onNinaExternalLink(): SDK fires this method when Nina VA does a handoff to application to display Application screens.
onDisplayed(): SDK fires this method when Chat is rendered and visible to user.
onError(): SDK fires this method when input field is disabled during the engagement preventing user from typing. Cases when input field is disabled are network disconnect, agent Left engagement, chat router timeout, engagement is denied etc
onCustomerMessage(): SDK fires this method every time customer send a message.
onVAMessage(): Allows application to listend to virtual agent message.
onEngagementCreated(): SDK fires this method when an engagement is created.
onEngagementEnded(): SDK fires this method when an engagement is ended.
onConversationResolved(): SDK fires this method when a conversation is resolved.
Handling Minimized state of engagement
User should be provided with a feedback of new agent messages when messaging window is minimized. Once the messaging window is minimized its application responsibility to query for new agent messaging within the application viewWillAppear method, as user navigates from one screen to another this code should be reexecuted for every screen user is in.
/**
You must initialize the GetMessageAPI in your viewController viewDidLoad method.
**/
override func viewDidLoad() {
super.viewDidLoad()
self.message = GetMessageAPI.getInstance();
}
/**
To continue receiving agent message while in minimized state and to display a restore button,
refer the following code snippet
**/
override func viewWillAppear(_ animated: Bool) {
// Check if there is an engagement session in progress
if(NuanMessaging.getInstance().getChatProgress()) {
//continue pooling for new agent messages , also can display a restore button here
self.message.getMessages({ (response, error) in
//If there is a new message from agent, handle it by showing a counter on the restore button or a notification prompt etc
//In case if agent closes the session , need to add the following code so that webview is notfied accordingly in case of hybrid engagement
//also app can hide the restore button and display chat live button.
if(response?.messageType == "chatLine") {
ChimeSound.sharedInstance.playSound()
}
else if(response?.messageType == "stateChange") {
let stateProp:String! = "state"
let stateText: String! = "display.text"
if(response?.getProperty(stateProp) == "closed") {
DispatchQueue.main.async {
self.webView.evaluateJavaScript("window.top.inqFrame.Inq.FlashPeer.closeChat()", completionHandler: nil)
}
}
}
})
}
}
override func viewWillDisappear(_ animated: Bool) {
//Very important to cancel the GetMessage pooling when viewController is about to disappear and moves to new viewController
if(NuanMessaging.getInstance().getChatProgress()) {
message.cancelGetMessages()
}
}
Plain Bubble View in Converstation Screen
Plain bubble Converstation View along with an optional Icon for agent is the default view displayed for Messaging UI.
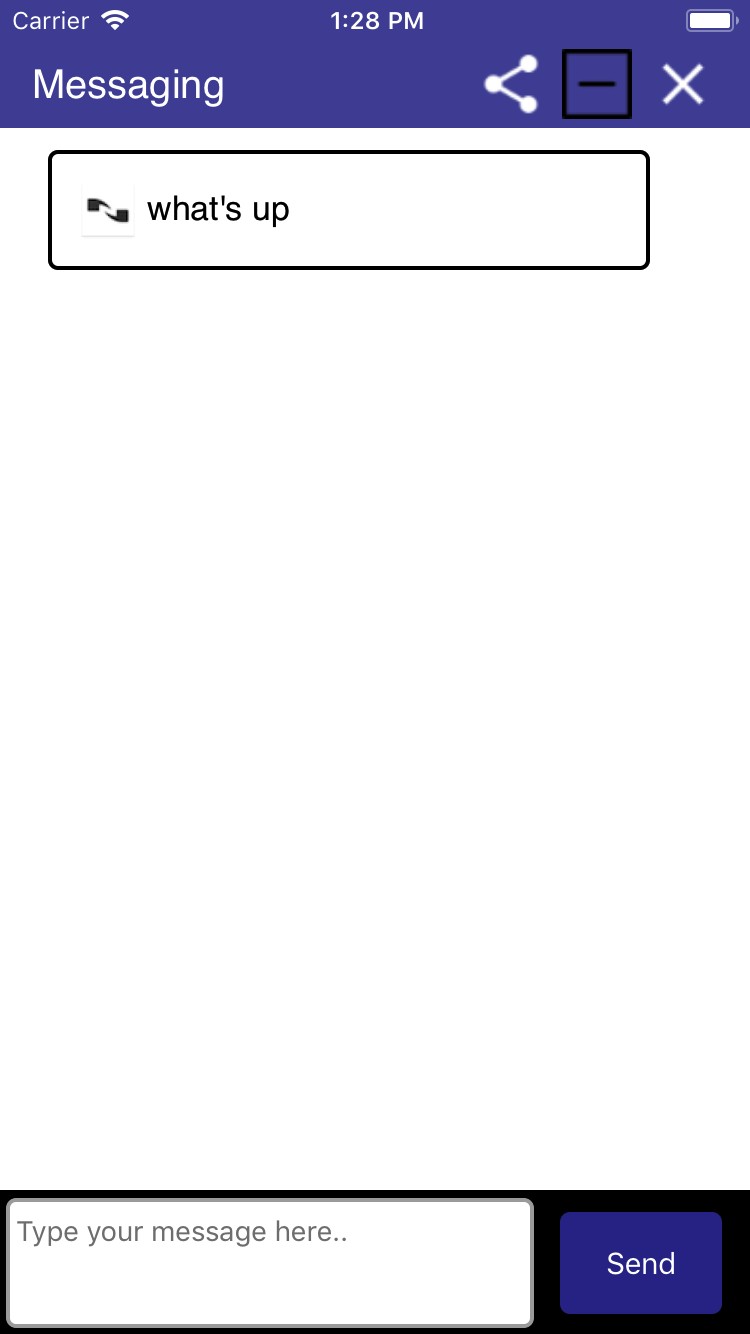
Styling Send Button
Properties to customize send button is exposed from SendButtonProperties class. Application has to pass an instance of this class having modified properties into the framework.
public func setSendButtonProperties(properties: SendButtonProperties)
Following properties in SendButtonProperties lets you change the look and feel of Send Button.
Name | type | default | description |
---|---|---|---|
borderColor | UIColor | UIColor.white |
Use this property to change the send button border color |
borderWidth | Int | 0 | Use this property to change the border width. |
cornerRadius | Int | 5 | Use this property to change the corner Radius. |
buttonTitle | String | Send | Lets you change the Send button label. |
backgroundColor | UIColor | UIColor(red: 50.0/255.0, green: 53.0/255.0, blue: 149.0/255.0, alpha: 1.0) | Change background color of send button. |
textColor | UIColor | UIColor.white | Change the send button label foreground color. |
gradientEffect | [UIColor] | [UIColor.white, UIColor.white, UIColor.white] | Lets you add a gradient effect to button. |
gradientEffectLocations | [NSNumber] | [0.0, 0.0, 0.0] | Lets you add the location of gradient effects. |
useGradientEffect | Bool | false | you must change it to true to have the gradient take effect. |
buttonImageData | Data | Nil | Lets you set an image as the background to send button. buttonTitle won't have any effect when you set an Image. |
disableImageData | Data | Nil | Lets you set an image as the background to send button.This will be displayed when SDK disables send button |
buttonWidth | Int | 80 | Lets you set the width of send button. |
buttonFieldRightMargin | int | 14 | Lets you set the send button right margin |
buttonFieldTopMargin | int | 4 | Lets you set the send button top margin |
buttonFieldBottomMargin | int | 3 | Lets you set the send button bottom margin |
buttonHeight | int | 0 | Lets you set the height of button image, setting button height make the top an bottom margin ineffective |
toggleSendStateAfterMsgSend | boolean | false | Set the send button to disabled state when Customer Text input contains 0 chars. Button will move to enabled state as soon as customer start typing. |
Styling Customer Text Input Field
Properties to customize Customer Input field is exposed from CustomerInputFieldProperties class. Application has to pass an instance of this class having modified properties into the framework.
public func setCustomerInputProperties(properties: CustomerInputFieldProperties)
Following properties in CustomerInputFieldProperties lets you change the look and feel of Input Field.
Name | type | default | description |
---|---|---|---|
hintText | String | Type your message here.. |
Lets you change the hint text displayed in the input field. |
borderColor | UIColor | UIColor.white | Use this property to change the border color. |
borderWidth | Int | 0 | Use this property to change the border width. |
cornerRadius | Int | 5 | Use this property to change the corner Radius. |
inputMessageBackgroundColor | UIColor | UIColor.white | Change background color of Input Field. |
textColor | UIColor | UIColor.gray | Change text foreground color. |
placeHolderColor | UIColor | UIColor.gray | Lets you change the hinttext foreground color. |
containerBackgroundColor | UIColor | UIColor.gray | Lets you change the background color of the container where input text field and Send button is displayed. |
dismissKeyBoardOnReturn | boolean | true | Lets you control the action that happens when user press return key. |
sendMsgOnReturn | boolean | false | Lets application control if message need to send when pressing return key on keyboard. |
inputFieldLeftMargin | int | 3 | Lets you set the input field left margin value. |
inputFieldRightMargin | int | 13 | Lets you set the input field right margin. |
inputFieldTopMargin | int | 4 | Lets you set the input field top margin. |
inputFieldBottomMargin | int | 3 | Lets you set the input field bottom inputFieldTopMargin. |
textFieldFont | UIFont | UIFont(name: kFontName, size: 14.0) | Lets you change the input field font size. |
textFieldTopPadding | Int | 0 | Lets you change the input field top padding. |
textFieldLeftPadding | Int | 0 | Lets you change the input field left padding. |
textFieldBottomPadding | Int | 0 | Lets you change the input field bottom padding. |
textFieldRightPadding | Int | 0 | Lets you change the input field right padding. |
keyboardType | UIKeyboardType | UIKeyboardType.default | Lets you change the input keyboard type. |
isCharacterLimitRequired![]() |
bool | false | lets you configure the display of character limit view when user engaged with VA. |
limitDisplayThreshold | int | 100 | lets you configure the threshold at which character limit view displays. |
characterLimitForVA | int | 120 | Maximum character that can be entered |
characterLimitBottomPadding | int | 5 | Character limit view bottom padding |
characterLimitRightPadding | int | 5 | Character limit view right padding |
characterLimitTopPadding | int | 5 | Character limit view top padding |
characterLimitViewWidth | int | 28 | Character limit view width |
characterLimitTextColor | UIColor | gray | Character limit view text color |
characterLimitTextFont | UIFont | UIFont(name: "Helvetica", size: 8) | Character limit view font style |
inputTextAlignment | NSTextAlignment | NSTextAlignment.left | Allows application to set the input text alignment |