Quick Start Guide
Prerequisites
Use of the Nuance Messaging SDK for iOS requires the following:
- Nuance CocoaPods Aartifactory PAT
- NuanceMessaging Pods or
- NuanceMessaging xcFrameworks
- Third party dependencies, mentioned below
- Xcode 8 and above
Get the SDK using CocoaPods
Contact your Nuance Client Services Manager to get the CocoaPods Artifactory Personal Access Token (PAT).
-
1. Configure CocoaPods Azure Universal Packages plugin
The CocoaPods plugin is used to download pods published as Universal Packages in Azure Artifacts feeds.
- Install the CocoaPods packages.
gem install ”cocoapods-azure-universal-packages”
- Install the CocoaPods packages.
-
2. Configure Azure CLI
The Azure CLI (Command-Line Interface) is a set of commands used to create and manage Azure resources.
- Open a Powershell terminal instance.
- Install homebrew, if not already installed.
install homebrew
- Execute command:
brew update && brew install azure-cli
- Execute command:
az devops login
- Enter the PAT provided by Nuance Client Services Manager.
-
3. Enable the pod in your application
Note: This step can be ignored if your application is already pod enabled.
- Navigate to your application's source code folder.
- Execute command
pod init
Configure the SDK using CocoaPods
-
1. Configure the pod file
- Add the following line at the start of the podfile:
plugin 'cocoapods-azure-universal-packages', { :organization => 'https://dev.azure.com/nuance-ent-rd-fe' }
- Add the following as a pod dependency:
$nuanceVersion = '10.4.0' pod 'NuanceMessagingSDK', :http => 'https://dev.azure.com/nuance-ent-rd-fe/sdk-ios/_apis/packaging/feeds/nuance-sdk-ios/upack/packages/nuancemessagingsdk/versions/'+$nuanceVersion
- Add the following line at the start of the podfile:
-
2. Install third-party dependencies
- pod 'Alamofire'
- pod ‘AFNetworking’, ‘~>4.0.1’
- pod ‘JSONModel’
- pod ‘SVProgressHUD’
-
3. Execute command
pod install
Get the SDK as a zip from CocoaPods
The homebrew CLI tool can be downloaded here:
To download artifacts as a zip file, ensure that the gem version on the system is greater than 3.3.6.
Arguments: -v artifact version (mandatory) -f feed name (optional) (default value: nuance-sdk-ios) -d download folder path (optional) (default value: Tool directory) -p Azure devops PAT (optional) (default value: Tool will prompt for PAT at runtime, if not found) Examples: Navigate to downloaded directory in a terminal 1. To download SDK version "10.0.0" from Prod. ./aad.sh -v "10.0.0" Note: Tool will prompt for PAT at runtime, if not found. 2. To download SDK version "10.0.0" from different feed for example "nuance-sdk-ios-beta". ./aad.sh -v "10.0.0-beta.3" -f "nuance-sdk-ios-beta" Note: Tool will prompt for PAT at runtime, if not found. 3. To download SDK version "10.0.0" from different feed for example "nuance-sdk-ios-beta" and save it some other directory. ./aad.sh -v "10.0.0-beta.3" -f "nuance-sdk-ios-beta" -d "/path/to/download/folder" Note: Tool will prompt for PAT at runtime, if not found. 4. To download SDK version "10.0.0" using different PAT. ./aad.sh -v "10.0.0" -p "YOUR_PAT"
-
NuanceGuide.Framework
NuanceGuide.Framework adds support for rich media capabilities to Nuance Message.
Get Nuance Messaging iOS SDK
Contact Nuance support to get the iOS SDK package.
Note: This distribution mechanism will not be supported in the future. Nuance recommends the use of CocoaPods artifactory, instead.The package is comprised of the following items:
-
NuanceMessagingCore: This framework comprises the Core Messaging APIs mentioned in this document.
NuanceMessagingUI: This Framework exposes Messaging and Call UI. Also exposes properties to brand the UI layer.
NinaCobra:ASR/TTS engine to recognize user speach input and provide Text to Speach functionality in your Messaging UI.
-
NuanceGuide.Framework
NuanceGuide.Framework adds support for rich media capabilities to Nuance Message.
Configure Xcode project settings to add Nuance Messaging Frameworks
Follow the following steps to configure the Xcode project settings to use the NuanceMessaging frameworks.
Note: This distribution mechanism will not be supported in the future. Nuance recommends the use of CocoaPods artifactory, instead.-
Link the given NuanceMessagingCore.xcframework to Xcode
- Navigate to the General tab in the Xcode project settings window.
- Expand Embeded binaries subsection.
- Press the plus button and select the provided NuanceMessagingCore.framework file from the file browser window.
-
Link the given NuanceMessagingUI.xcframework to Xcode
- Navigate to the General tab in the Xcode project settings window.
- Expand the Embeded binaries subsection.
- Press the plus button and select the provided NuanceMessagingUI.framework file from the file browser window.
-
Link the given NinaCobra.xcframework to Xcode
- Navigate to the General tab in the Xcode project settings window.
- Expand the Embeded binaries subsection.
- Press the plus button and select the provided NinaCobra.framework file from the file browser window.
-
Import the frameworks to your workspace
- import NuanceMessagingUI
- import NuanceMessagingCore
- import NinaCobra
-
Add SDK dependencies
- AFNetworking.framework
Configure Xcode project settings to add Nuance Guide
First, follow the steps in the previous section to add NuanceGuide.framework to your workspace.
Note: This distribution mechanism will not be supported in the future. Nuance recommends the use of CocoaPods artifactory, instead.-
Import framework to your workspace
- import NuanceGuide
-
Add SDK dependencies
- Alamofire.framework
- AlamofireImage.framework
- SVProgressHUD.framework
- JSONModel.framework
Third-party licenses
Initialize messaging SDK module
You need to initialize the SDK before doing any operation with it. Either at startup, or at the desired point in your application flow, initialize the SDK with necessary parameters.
To initialize the module, invoke the initialize method in the NuanceMessaging class.
NuanceMessaging
- initialize:(NSString *) dataCenter andClientID:(NSString*) clientID andClientSecret:(NSString*) clientSecret
- initialize:(NSString *) dataCenter andClientID:(NSString*) clientID andClientSecret:(NSString*) clientSecret andTagServerName:(NSString*) tagServerName
- initialize:(NSString *) dataCenter andClientID:(NSString*) clientID andClientSecret:(NSString*) clientSecret andTagServerName:(NSString*) tagServerName andFCMToken:(NSString*) fcmToken
dc | Name of the data center where your messaging session is processed. |
clientID | Pass the Nuance provided clientID which will be used identify your messaging Instance. |
clientSecret | Pass the Nuance provided clientSecret code. |
tagServerName | Request Nuance to provide the tagserver used for handling your messages. |
fcmToken | If a device registration token is provided, the SDK can register to receive Push Messages from Nuance Messaging System. |
class AppDelegate: UIResponder, UIApplicationDelegate { var window: UIWindow? func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool { // Override point for customization after application launch. let clientID = "Your client Id"; let cientSecret = "your client secret" let nuanMessaging = NuanMessaging.getInstance(); nuanMessaging?.initialize("west", andClientID:clientID, andClientSecret:clientSecret, andTagServerName:"bestbrands") return true } }
Messaging window launch/restore flow diagram
Your application needs to follow the code path below when a user launches or navigates to different screens within the app.
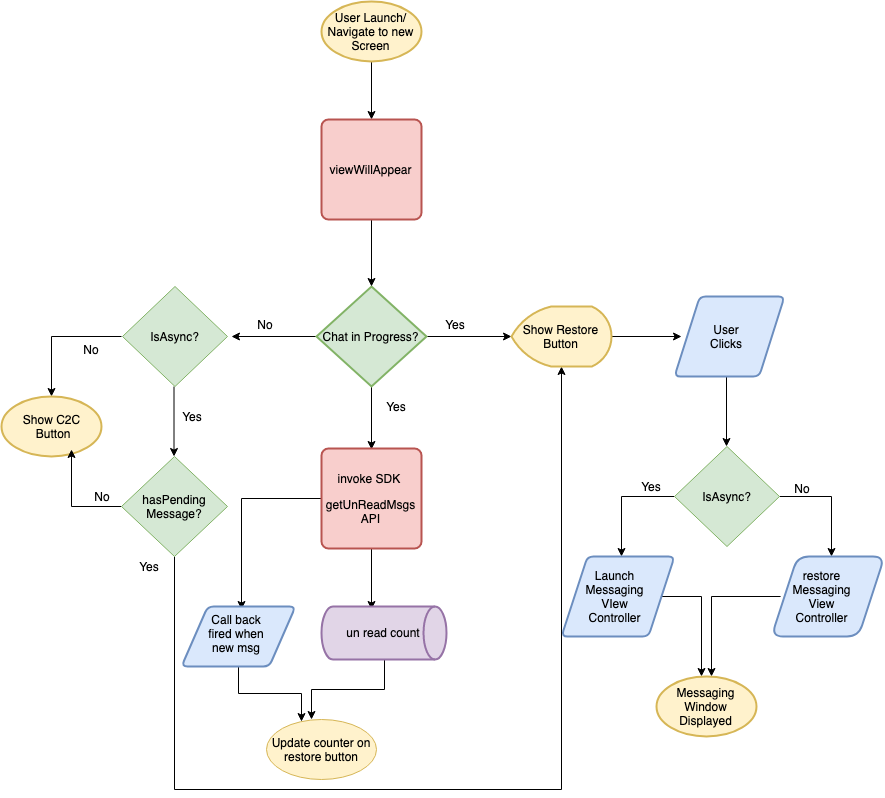